适配器模式(Adapter Design Pattern)
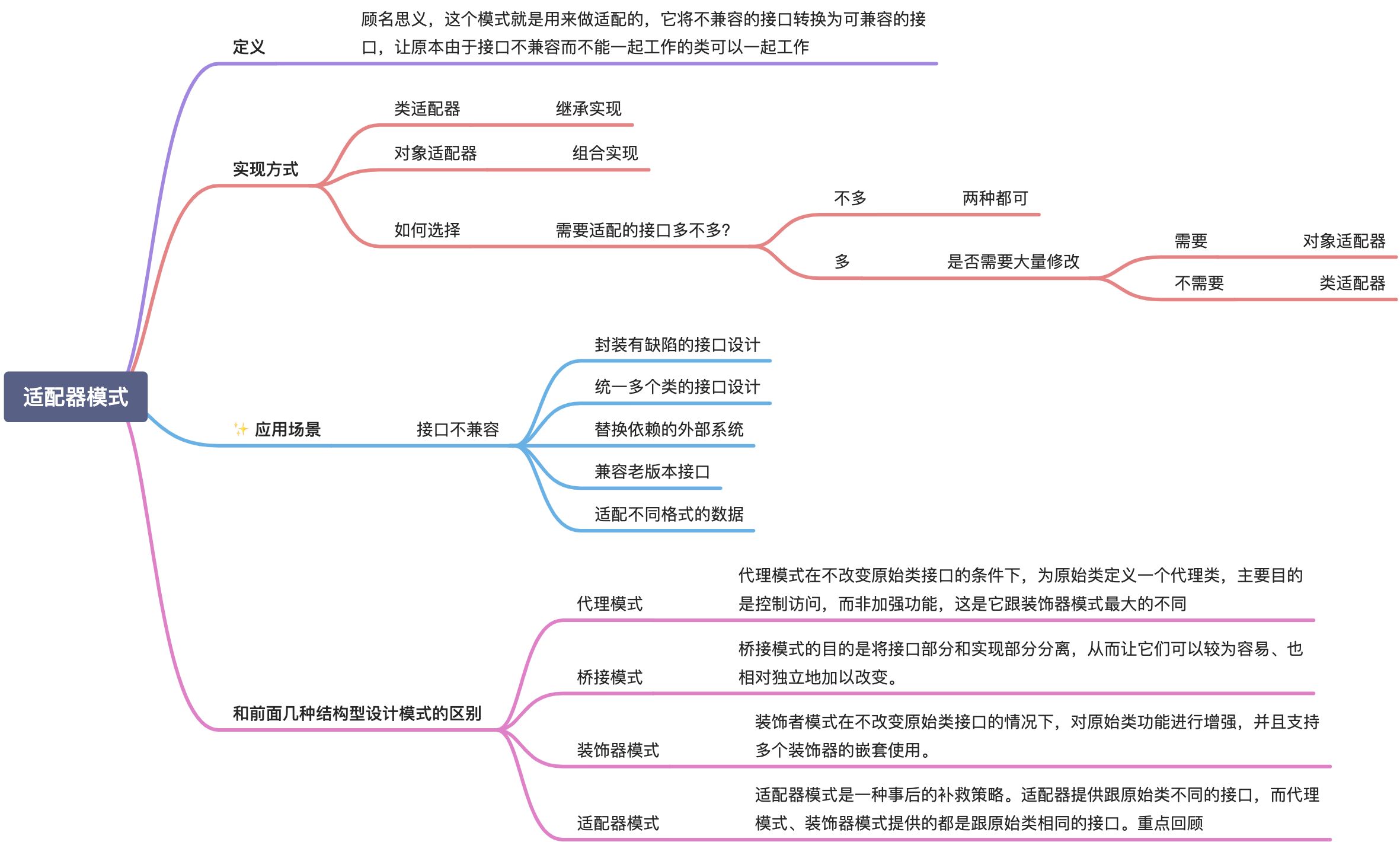
定义
适配器模式用来进行接口适配,它将不兼容的接口转化为可兼容的接口,让原本不兼容不能一起工作的类可以一起工作
实现方式
- 类适配器–继承实现
- 对象适配器–组合实现
应用场景
- 封装有缺陷的接口设计
- 同一多个类的接口设计
- 替换依赖的外部系统
- 兼容老版本接口
- 适配不同格式的数据
go源码实现
假设我现在有一个运维系统,需要分别调用阿里云和 AWS 的 SDK 创建主机,两个 SDK 提供的创建主机的接口不一致,此时就可以通过适配器模式,将两个接口统一。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
| package adapter
import "fmt"
type ICreateServer interface { CreateServer(cpu, mem float64) error }
type AWSClient struct{}
func (c *AWSClient) RunInstance(cpu, mem float64) error { fmt.Printf("aws client run success, cpu: %f, mem: %f", cpu, mem) return nil }
type AwsClientAdapter struct { Client AWSClient }
func (a *AwsClientAdapter) CreateServer(cpu, mem float64) error { a.Client.RunInstance(cpu, mem) return nil }
type AliyunClient struct{}
func (c *AliyunClient) CreateServer(cpu, mem int) error { fmt.Printf("aws client run success, cpu: %d, mem: %d", cpu, mem) return nil }
type AliyunClientAdapter struct { Client AliyunClient }
func (a *AliyunClientAdapter) CreateServer(cpu, mem float64) error { a.Client.CreateServer(int(cpu), int(mem)) return nil }
|